A Beginner’s Guide to JavaScript: Learn the Fundamentals
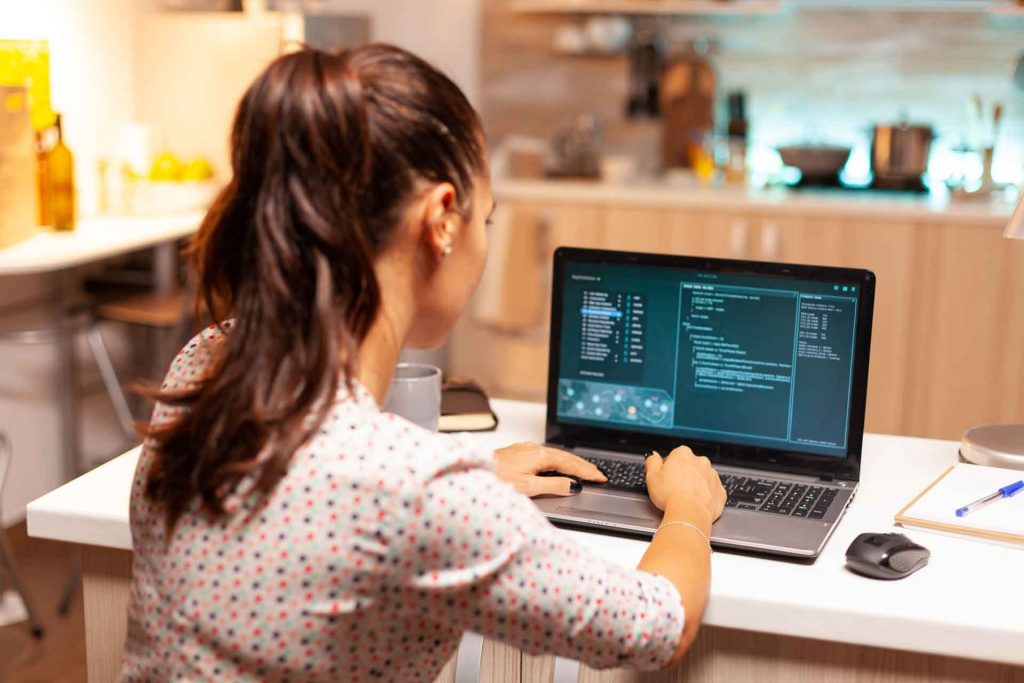
JavaScript is a popular programming language used to build interactive web applications. As a beginner, learning JavaScript can be a daunting task. However, with the right resources and a willingness to learn, you can master the fundamentals of this language.
In this blog, we will go over the basics of JavaScript, including variables, data types, functions, and loops. By the end of this guide, you should have a solid foundation in JavaScript and be ready to tackle more complex programming concepts.
1) Variables
In JavaScript, variables are used to store values that can be used later in the code. To declare a variable, you must use the “var,” “let,” or “const” keyword. For example:
var myVariable = “Hello World!”;
let myOtherVariable = 42;
const myConstantVariable = true;
2) Data Types
JavaScript has several data types, including strings, numbers, booleans, null, and undefined. To determine the type of a variable, you can use the “typeof” operator. For example:
typeof “Hello World!”; // returns “string”
typeof 42; // returns “number”
typeof true; // returns “boolean”
typeof null; // returns “object”
typeof undefined; // returns “undefined”
3) Functions
Functions are a fundamental part of JavaScript. They allow you to group a set of statements together and execute them whenever you want. To create a function, you must use the “function” keyword, followed by the function name and parameters. For example:
function sayHello(name) {
console.log(“Hello, ” + name + “!”);
}
sayHello(“John”); // prints “Hello, John!”
4) Loops
Loops are used to execute a set of statements multiple times. In JavaScript, there are two types of loops: “for” and “while.” The “for” loop is used when you know how many times you want to execute the statements. The “while” loop is used when you don’t know how many times you want to execute the statements. For example:
for (let i = 0; i < 10; i++) {
console.log(i);
}
let j = 0;
while (j < 10) {
console.log(j);
j++;
}
In conclusion,
JavaScript is an essential language for web development, and mastering the fundamentals is crucial for success. By understanding variables, data types, functions, and loops, you will be well on your way to becoming a proficient JavaScript programmer. With practice and dedication, you can unlock the full potential of this powerful programming language.
For inquires : Click here
Read more blogs: Click here
Featured image source: Image by DCStudio on Freepik